이 페이지는 Visual Basic 애플리케이션 내에서 Windows 텍스트 음성 변환 음성을 사용하는 방법에 대한 자습서를 제공합니다.
Visual Basic 2008 Express Edition 이라는 뛰어난 Visual Basic .NET 무료 버전은 MSDN 웹 사이트에서 다운로드할 수 있습니다.
.NET System.Speech 구성 요소 에 대한 전체 설명서입니다 .
1. "안녕하세요!" 신청
응용 프로그램에서 "안녕하세요"라고 말하는 것은 매우 쉽습니다. 콘솔 응용 프로그램을 만들고 Project|Add Reference를 사용하여 System.Speech 구성 요소에 대한 참조를 추가합니다.
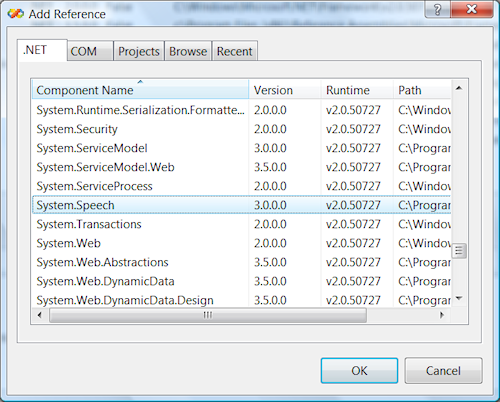
그런 다음 모듈 소스에 다음 줄을 추가합니다.
Imports System.Speech.Synthesis
Module Module1
Sub Main()
Dim synth As New SpeechSynthesizer
synth.Speak("Hello world from the default voice.")
End Sub
End Module
|
2. 사용 가능한 음성 열거
사용 가능한 각 음성이 인사하는 Hello World의 정교함:
Imports System.Speech.Synthesis
Module Module1
Sub Main()
Dim synth As New SpeechSynthesizer
Dim voices = synth.GetInstalledVoices()
For Each v As InstalledVoice In voices
System.Console.WriteLine(v.VoiceInfo.Name)
synth.SelectVoice(v.VoiceInfo.Name)
synth.Speak("Hello from " & v.VoiceInfo.Name)
Next
End Sub
End Module
|
3. SSML을 사용하여 단어를 말하는 방식 수정
SpeakSSML() 함수를 사용하면 음성 합성 마크업 언어 를 사용하여 말할 텍스트를 마크업할 수 있습니다 . 다음은 간단한 예입니다.
Imports System.Speech.Synthesis
Module Module1
Sub Main()
Dim synth As New SpeechSynthesizer
Dim txt As String
txt = "<speak version='1.0' xml:lang='en-GB'>"
txt &= "One potato, two potato, three potato, four. "
txt &= "<prosody rate='-50%'>"
txt &= "One potato, two potato, three potato, four. "
txt &= "</prosody></speak>"
synth.SpeakSsml(txt)
End Sub
End Module
|
이 코드는 영국 영어 음성(en-GB)이 있을 것으로 예상하고 미국 영어 음성만 있는 경우 en-US를 사용합니다.
4. 단계별 텍스트 프롬프트 구축
SSML 마크업을 사용할 필요 없이 PromptBuilder 객체를 사용하여 복잡한 프롬프트를 하나씩 빌드할 수 있습니다. 예를 들어:
Imports System.Speech.Synthesis
Imports System.Globalization
Module Module1
Sub Main()
Dim synth As New SpeechSynthesizer
Dim myPrompt = New PromptBuilder(New CultureInfo("en-US"))
Dim mainStyle = New PromptStyle(PromptRate.Medium)
Dim slowStyle = New PromptStyle(PromptRate.Slow)
myPrompt.StartStyle(slowStyle)
myPrompt.AppendText("Better")
myPrompt.AppendTextWithPronunciation("Reading", "riːdɪŋ")
myPrompt.EndStyle()
myPrompt.StartStyle(mainStyle)
myPrompt.AppendText("is a book published by the University of")
myPrompt.AppendTextWithPronunciation("Reading.", "rɛdɪŋ")
myPrompt.AppendBreak()
myPrompt.AppendText("The acronym")
myPrompt.AppendTextWithHint("BBC", System.Speech.Synthesis.SayAs.SpellOut)
myPrompt.AppendText("stands for the British Broadcasting Corporation.")
myPrompt.AppendBreak()
myPrompt.EndStyle()
synth.Speak(myPrompt)
End Sub
End Module
|